OAuth U2M in a nutshell
Published: November 18, 2024
The OAuth U2M in a nutshell guide presents the OAuth 2.0 User To Machine authentication process in a simplified way. It dynamically provides various sample codes. The stepper below shows the various steps, which are clickable:
Step 1 - Create an application
Before you can use Orange APIs, there are various steps that are needed to get you started on the Orange Developer portal. These steps are described in: How to start with Orange Developer.
For the authentication process, you need:
- Have your `client_id` and `client_secret` readily available as they are used to identify your application and enable API call.
- You will also need the base URL of the Orange API you wish to access. You can find it in the API Reference documentation. Note: in this guide we will use `api.orange.com` as an example.
Below is a diagram of the various steps for the OAuth 2.0 authentication process:
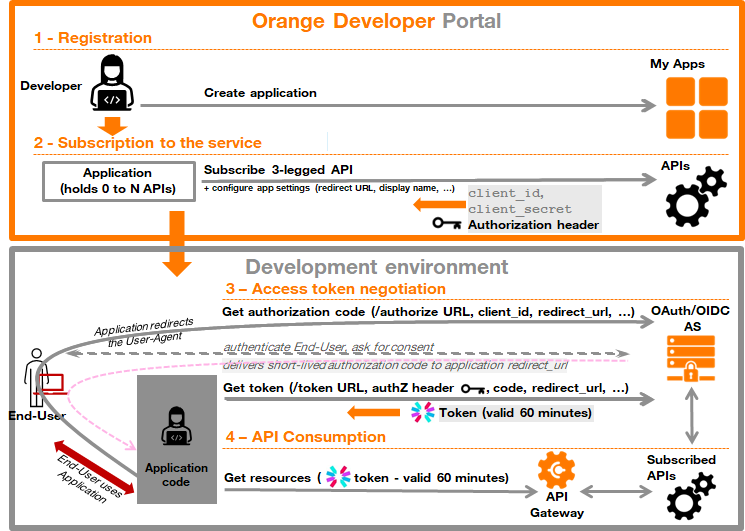
Step 2 - Request the authorization code
1. Construct an authorization code request URL with the following mandatory parameters:
- response_type=code. This is fixed value
- client_id. This is provided by Orange when creating your app
- the list of API scopes your application requires. Individual scopes should be separated by whitespace, and the whole line URL-encoded. This information is available in the API documentation, section Getting Started
- redirect_uri, i.e. the URL on which your application wishes to receive the authorization code. This URL must be registered beforehand under the config section of your application on the Orange Developer portal
- state, i.e. an opaque value used by the client to maintain state between the authorization code request and the callback. The authorization server includes this value when redirecting the user-agent back to the client. This parameter SHOULD be used for preventing cross-site request forgery
Example URL:
https://api.orange.com/openidconnect/fr/v1/authorize?response_type=code&client_id=YOUR_CLIENT_ID&scope=SCOPE&redirect_uri=YOUR_REDIRECT_URI&state=YOUR_STATE
2. Direct the API end-users to this constructed URI using HTTP redirection responses, or by other means available to it via the user-agent. This way, the end users will be in direct contact with the authorization server and will be able to grant the access request.
Step 3 - Request the OAuth access token by exchanging it against the authorization code
1. Assuming the API end users grant access, the authorization server redirects the user-agent back to your redirection URI provided earlier. The redirection URI includes an authorization code and any local state you provided earlier.
2. Send a POST request to the token endpoint with the following parameters, cf example below:
- authorization_header: your application credentials encoded in base64
- grant_type=authorization_code. Fixed value
- code: the authorization code received
- redirect_uri: must be the same as the one used in the authorization request and declared in Orange Developer
curl -X POST \ -H "Authorization: Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET" \ -d "grant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI" \ https://api.orange.com/openidconnect/fr/v1/token
Example cURL
<?php
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.orange.com/openidconnect/fr/v1/token');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST');
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET',
'Content-Type: application/x-www-form-urlencoded',
]);
curl_setopt($ch, CURLOPT_POSTFIELDS, 'grant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI');
$response = curl_exec($ch);
curl_close($ch);
using System.Net.Http;
using System.Net.Http.Headers;
HttpClient client = new HttpClient();
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Post, "https://api.orange.com/openidconnect/fr/v1/token");
request.Headers.Add("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET");
request.Content = new StringContent("grant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI");
request.Content.Headers.ContentType = new MediaTypeHeaderValue("application/x-www-form-urlencoded");
HttpResponseMessage response = await client.SendAsync(request);
response.EnsureSuccessStatusCode();
string responseBody = await response.Content.ReadAsStringAsync();
import java.io.IOException
import okhttp3.FormBody
import okhttp3.OkHttpClient
import okhttp3.Request
val client = OkHttpClient()
val formBody = FormBody.Builder()
.add("grant_type", "authorization_code")
.add("code", "AUTHORIZATION_CODE")
.add("redirect_uri", "YOUR_REDIRECT_URI")
.build()
val request = Request.Builder()
.url("https://api.orange.com/openidconnect/fr/v1/token")
.post(formBody)
.header("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET")
.header("Content-Type", "application/x-www-form-urlencoded")
.build()
client.newCall(request).execute().use { response ->
if (!response.isSuccessful) throw IOException("Unexpected code $response")
response.body!!.string()
}
extern crate reqwest;
use reqwest::header;
fn main() -> Result<(), Box<dyn std::error::Error>> {
let mut headers = header::HeaderMap::new();
headers.insert("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET".parse().unwrap());
headers.insert("Content-Type", "application/x-www-form-urlencoded".parse().unwrap());
let client = reqwest::blocking::Client::builder()
.redirect(reqwest::redirect::Policy::none())
.build()
.unwrap();
let res = client.post("https://api.orange.com/openidconnect/fr/v1/token")
.headers(headers)
.body("grant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI")
.send()?
.text()?;
println!("{}", res);
Ok(())
}
Example fetch
fetch('https://api.orange.com/openidconnect/fr/v1/token', {
method: 'POST',
headers: {
'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET'
},
body: new URLSearchParams({
'grant_type': 'authorization_code',
'code': 'AUTHORIZATION_CODE',
'redirect_uri': 'YOUR_REDIRECT_URI'
})
});
Example jQuery
$.ajax({
url: 'https://api.orange.com/openidconnect/fr/v1/token',
crossDomain: true,
method: 'post',
headers: {
'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET'
},
contentType: 'application/x-www-form-urlencoded',
data: {
'grant_type': 'authorization_code',
'code': 'AUTHORIZATION_CODE',
'redirect_uri': 'YOUR_REDIRECT_URI'
}
}).done(function(response) {
console.log(response);
});
Example XHR
const data = new URLSearchParams({
'grant_type': 'authorization_code',
'code': 'AUTHORIZATION_CODE',
'redirect_uri': 'YOUR_REDIRECT_URI'
});
let xhr = new XMLHttpRequest();
xhr.withCredentials = true;
xhr.open('POST', 'https://api.orange.com/openidconnect/fr/v1/token');
xhr.setRequestHeader('Authorization', 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET');
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
xhr.onload = function() {
console.log(xhr.response);
};
xhr.send(data);
Example HttpClient
import java.io.IOException;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpRequest.BodyPublishers;
import java.net.http.HttpResponse;
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.orange.com/openidconnect/fr/v1/token"))
.POST(BodyPublishers.ofString("grant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI"))
.setHeader("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET")
.setHeader("Content-Type", "application/x-www-form-urlencoded")
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
Example HttpURLConnection
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStreamWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Scanner;
class Main {
public static void main(String[] args) throws IOException {
URL url = new URL("https://api.orange.com/openidconnect/fr/v1/token");
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
httpConn.setRequestMethod("POST");
httpConn.setRequestProperty("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET");
httpConn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
httpConn.setDoOutput(true);
OutputStreamWriter writer = new OutputStreamWriter(httpConn.getOutputStream());
writer.write("grant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI");
writer.flush();
writer.close();
httpConn.getOutputStream().close();
InputStream responseStream = httpConn.getResponseCode() / 100 == 2
? httpConn.getInputStream()
: httpConn.getErrorStream();
Scanner s = new Scanner(responseStream).useDelimiter("\A");
String response = s.hasNext() ? s.next() : "";
System.out.println(response);
}
}
Example jsop
import java.io.File; import java.io.FileInputStream; import java.io.IOException; import org.jsoup.Connection; import org.jsoup.Jsoup; class Main { public static void main(String[] args) throws IOException { Connection.Response response = Jsoup.connect("https://api.orange.com/openidconnect/fr/v1/token") .header("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET") .header("Content-Type", "application/x-www-form-urlencoded") .requestBody("grant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI") .method(org.jsoup.Connection.Method.POST) .ignoreContentType(true) .execute(); System.out.println(response.parse()); } }
Example okHttp
import java.io.IOException;
import okhttp3.FormBody;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
OkHttpClient client = new OkHttpClient();
RequestBody formBody = new FormBody.Builder()
.add("grant_type", "authorization_code")
.add("code", "AUTHORIZATION_CODE")
.add("redirect_uri", "YOUR_REDIRECT_URI")
.build();
Request request = new Request.Builder()
.url("https://api.orange.com/openidconnect/fr/v1/token")
.post(formBody)
.header("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET")
.header("Content-Type", "application/x-www-form-urlencoded")
.build();
try (Response response = client.newCall(request).execute()) {
if (!response.isSuccessful()) throw new IOException("Unexpected code " + response);
response.body().string();
}
Example node-fetch
import fetch from 'node-fetch';
fetch('https://api.orange.com/openidconnect/fr/v1/token', {
method: 'POST',
headers: {
'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET',
},
body: new URLSearchParams({
'grant_type': 'authorization_code'
'code': 'AUTHORIZATION_CODE',
'redirect_uri': 'YOUR_REDIRECT_URI'
})
});
Example http
import https from 'https';
const options = {
hostname: 'api.orange.com',
path: '/openidconnect/fr/v1/token',
method: 'POST',
headers: {
'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET',
'Content-Type': 'application/x-www-form-urlencoded',
}
};
const req = https.request(options, function (res) {
const chunks = [];
res.on('data', function (chunk) {
chunks.push(chunk);
});
res.on('end', function () {
const body = Buffer.concat(chunks);
console.log(body.toString());
});
});
req.write(new URLSearchParams({
'grant_type': 'authorization_code',
'code': 'AUTHORIZATION_CODE',
'redirect_uri': 'YOUR_REDIRECT_URI'
}).toString());
req.end();
Example requests
import requests
headers = {
'Authorization': 'BASE64_ENCODED_CLIENT_ID_AND_SECRET',
'Content-Type': 'application/x-www-form-urlencoded',
}
data = {
'grant_type': 'authorization_code',
'code': 'AUTHORIZATION_CODE',
'redirect_uri': 'YOUR_REDIRECT_URI',
}
response = requests.post('https://api.orange.com/openidconnect/fr/v1/token', headers=headers, data=data)
Example http.client
import http.client
conn = http.client.HTTPSConnection('api.orange.com')
headers = {
'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET',
'Content-Type': 'application/x-www-form-urlencoded',
}
conn.request(
'POST',
'/openidconnect/fr/v1/token',
'grant_type=authorization_code&code=AUTHORIZATION_CODE&redirect_uri=YOUR_REDIRECT_URI',
headers
)
response = conn.getresponse()
3. On success, you will receive a response with an `access_token` to use for accessing protected resources, for instance:
json { "token_type": "Bearer", "access_token": "YOUR_NEW_ACCESS_TOKEN", "expires_in": 3600, "refresh_token": "YOUR_NEW_REFRESH_TOKEN" }
Step 4 - Refresh the OAuth access token (if necessary)
If you have a `refresh_token`, you can use it to obtain a new `access_token` by sending a POST request to the token endpoint with the following parameters, cf exemple below:
- `authorization_header` (your application credentials encoded in base64)
- `grant_type=refresh_token`
- `refresh_token` (the refresh token obtained earlier)
curl -X POST \ -H "Authorization: Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET" \ -d "grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN" \ https://api.orange.com/openidconnect/fr/v1/token
Example cURL
<?php $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'https://api.orange.com/openidconnect/fr/v1/token'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'POST'); curl_setopt($ch, CURLOPT_HTTPHEADER, [ 'Authorization: Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET', 'Content-Type: application/x-www-form-urlencoded', ]); curl_setopt($ch, CURLOPT_POSTFIELDS, 'grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN'); $response = curl_exec($ch); curl_close($ch);
using System.Net.Http; using System.Net.Http.Headers; HttpClient client = new HttpClient(); HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Post, "https://api.orange.com/openidconnect/fr/v1/token"); request.Headers.Add("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET"); request.Content = new StringContent("grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN"); request.Content.Headers.ContentType = new MediaTypeHeaderValue("application/x-www-form-urlencoded"); HttpResponseMessage response = await client.SendAsync(request); response.EnsureSuccessStatusCode(); string responseBody = await response.Content.ReadAsStringAsync();
import java.io.IOException import okhttp3.FormBody import okhttp3.OkHttpClient import okhttp3.Request val client = OkHttpClient() val formBody = FormBody.Builder() .add("grant_type", "refresh_token") .add("refresh_token", "YOUR_REFRESH_TOKEN") .build() val request = Request.Builder() .url("https://api.orange.com/openidconnect/fr/v1/token") .post(formBody) .header("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET") .header("Content-Type", "application/x-www-form-urlencoded") .build() client.newCall(request).execute().use { response -> if (!response.isSuccessful) throw IOException("Unexpected code $response") response.body!!.string() }
extern crate reqwest; use reqwest::header; fn main() -> Result<(), Box<dyn std::error::Error>> { let mut headers = header::HeaderMap::new(); headers.insert("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET".parse().unwrap()); headers.insert("Content-Type", "application/x-www-form-urlencoded".parse().unwrap()); let client = reqwest::blocking::Client::builder() .redirect(reqwest::redirect::Policy::none()) .build() .unwrap(); let res = client.post("https://api.orange.com/openidconnect/fr/v1/token") .headers(headers) .body("grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN") .send()? .text()?; println!("{}", res); Ok(()) }
Example fetch
fetch('https://api.orange.com/openidconnect/fr/v1/token', { method: 'POST', headers: { 'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET' }, body: new URLSearchParams({ 'grant_type': 'refresh_token', 'refresh_token': 'YOUR_REFRESH_TOKEN' }) });
Example jQuery
$.ajax({ url: 'https://api.orange.com/openidconnect/fr/v1/token', crossDomain: true, method: 'post', headers: { 'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET' }, contentType: 'application/x-www-form-urlencoded', data: { 'grant_type': 'refresh_token', 'refresh_token': 'YOUR_REFRESH_TOKEN' } }).done(function(response) { console.log(response); });
Example XHR
const data = new URLSearchParams({ 'grant_type': 'refresh_token', 'refresh_token': 'YOUR_REFRESH_TOKEN' }); let xhr = new XMLHttpRequest(); xhr.withCredentials = true; xhr.open('POST', 'https://api.orange.com/openidconnect/fr/v1/token'); xhr.setRequestHeader('Authorization', 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET'); xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded'); xhr.onload = function() { console.log(xhr.response); }; xhr.send(data);
Example HttpClient
import java.io.IOException; import java.net.URI; import java.net.http.HttpClient; import java.net.http.HttpRequest; import java.net.http.HttpRequest.BodyPublishers; import java.net.http.HttpResponse; HttpClient client = HttpClient.newHttpClient(); HttpRequest request = HttpRequest.newBuilder() .uri(URI.create("https://api.orange.com/openidconnect/fr/v1/token")) .POST(BodyPublishers.ofString("grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN")) .setHeader("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET") .setHeader("Content-Type", "application/x-www-form-urlencoded") .build(); HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
Example HttpURLConnection
import java.io.IOException; import java.io.InputStream; import java.io.OutputStreamWriter; import java.net.HttpURLConnection; import java.net.URL; import java.util.Scanner; class Main { public static void main(String[] args) throws IOException { URL url = new URL("https://api.orange.com/openidconnect/fr/v1/token"); HttpURLConnection httpConn = (HttpURLConnection) url.openConnection(); httpConn.setRequestMethod("POST"); httpConn.setRequestProperty("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET"); httpConn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded"); httpConn.setDoOutput(true); OutputStreamWriter writer = new OutputStreamWriter(httpConn.getOutputStream()); writer.write("grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN"); writer.flush(); writer.close(); httpConn.getOutputStream().close(); InputStream responseStream = httpConn.getResponseCode() / 100 == 2 ? httpConn.getInputStream() : httpConn.getErrorStream(); Scanner s = new Scanner(responseStream).useDelimiter("\\A"); String response = s.hasNext() ? s.next() : ""; System.out.println(response); } }
Example jsop
import java.io.File; import java.io.FileInputStream; import java.io.IOException; import org.jsoup.Connection; import org.jsoup.Jsoup; class Main { public static void main(String[] args) throws IOException { Connection.Response response = Jsoup.connect("https://api.orange.com/openidconnect/fr/v1/token") .header("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET") .header("Content-Type", "application/x-www-form-urlencoded") .requestBody("grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN") .method(org.jsoup.Connection.Method.POST) .ignoreContentType(true) .execute(); System.out.println(response.parse()); } }
Example okHttp
import java.io.IOException; import okhttp3.FormBody; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.RequestBody; import okhttp3.Response; OkHttpClient client = new OkHttpClient(); RequestBody formBody = new FormBody.Builder() .add("grant_type", "refresh_token") .add("refresh_token", "YOUR_REFRESH_TOKEN") .build(); Request request = new Request.Builder() .url("https://api.orange.com/openidconnect/fr/v1/token") .post(formBody) .header("Authorization", "Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET") .header("Content-Type", "application/x-www-form-urlencoded") .build(); try (Response response = client.newCall(request).execute()) { if (!response.isSuccessful()) throw new IOException("Unexpected code " + response); response.body().string(); }
Example node-fetch
import fetch from 'node-fetch'; fetch('https://api.orange.com/openidconnect/fr/v1/token', { method: 'POST', headers: { 'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET' }, body: new URLSearchParams({ 'grant_type': 'refresh_token', 'refresh_token': 'YOUR_REFRESH_TOKEN' }) });
Example http
import https from 'https'; const options = { hostname: 'api.orange.com', path: '/openidconnect/fr/v1/token', method: 'POST', headers: { 'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET', 'Content-Type': 'application/x-www-form-urlencoded' } }; const req = https.request(options, function (res) { const chunks = []; res.on('data', function (chunk) { chunks.push(chunk); }); res.on('end', function () { const body = Buffer.concat(chunks); console.log(body.toString()); }); }); req.write(new URLSearchParams({ 'grant_type': 'refresh_token', 'refresh_token': 'YOUR_REFRESH_TOKEN' }).toString()); req.end();
Example requests
import requests headers = { 'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET', 'Content-Type': 'application/x-www-form-urlencoded', } data = { 'grant_type': 'refresh_token', 'refresh_token': 'YOUR_REFRESH_TOKEN', } response = requests.post('https://api.orange.com/openidconnect/fr/v1/token', headers=headers, data=data)
Example http.client
import http.client conn = http.client.HTTPSConnection('api.orange.com') headers = { 'Authorization': 'Basic BASE64_ENCODED_CLIENT_ID_AND_SECRET', 'Content-Type': 'application/x-www-form-urlencoded', } conn.request( 'POST', '/openidconnect/fr/v1/token', 'grant_type=refresh_token&refresh_token=YOUR_REFRESH_TOKEN', headers ) response = conn.getresponse()
On success, you will receive a response with a `refresh_token`, for instance:
json { "token_type": "Bearer", "access_token": "YOUR_REFRESHED_ACCESS_TOKEN", "expires_in": 3600, }
Step 5 - Consume the API
Your application is now ready to consume the Orange API’s resources protected by the OAuth 2.0 U2M protocol.
For each API call to protected resources, include the `access_token` in the HTTP Authorization header as follows: `Authorization: Bearer {access_token}`, e.g.:
curl -X GET \ -H "Authorization: Bearer YOUR_ACCESS_TOKEN" \ https://api.orange.com/{api}/vM/{resource}
Example cURL
<?php $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, 'https://api.orange.com/{api}/vM/{resource}'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'GET'); curl_setopt($ch, CURLOPT_HTTPHEADER, [ 'Authorization: Bearer YOUR_ACCESS_TOKEN', ]); $response = curl_exec($ch); curl_close($ch);
using System.Net.Http; HttpClient client = new HttpClient(); HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Get, "https://api.orange.com/{api}/vM/{resource}"); request.Headers.Add("Authorization", "Bearer YOUR_ACCESS_TOKEN"); HttpResponseMessage response = await client.SendAsync(request); response.EnsureSuccessStatusCode(); string responseBody = await response.Content.ReadAsStringAsync();
import java.io.IOException import okhttp3.OkHttpClient import okhttp3.Request val client = OkHttpClient() val request = Request.Builder() .url("https://api.orange.com/{api}/vM/{resource}") .header("Authorization", "Bearer YOUR_ACCESS_TOKEN") .build() client.newCall(request).execute().use { response -> if (!response.isSuccessful) throw IOException("Unexpected code $response") response.body!!.string() }
extern crate reqwest; use reqwest::header; fn main() -> Result<(), Box<dyn std::error::Error>> { let mut headers = header::HeaderMap::new(); headers.insert("Authorization", "Bearer YOUR_ACCESS_TOKEN".parse().unwrap()); let client = reqwest::blocking::Client::builder() .redirect(reqwest::redirect::Policy::none()) .build() .unwrap(); let res = client.get("https://api.orange.com/{api}/vM/{resource}") .headers(headers) .send()? .text()?; println!("{}", res); Ok(()) }
Example fetch
fetch('https://api.orange.com/{api}/vM/{resource}', { headers: { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN' } });
Example jQuery
$.ajax({ url: 'https://api.orange.com/{api}/vM/{resource}', crossDomain: true, headers: { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN' } }).done(function(response) { console.log(response); });
Example XHR
let xhr = new XMLHttpRequest(); xhr.withCredentials = true; xhr.open('GET', 'https://api.orange.com/{api}/vM/{resource}'); xhr.setRequestHeader('Authorization', 'Bearer YOUR_ACCESS_TOKEN'); xhr.onload = function() { console.log(xhr.response); }; xhr.send();
Example HttpClient
import java.io.IOException; import java.net.URI; import java.net.http.HttpClient; import java.net.http.HttpRequest; import java.net.http.HttpResponse; HttpClient client = HttpClient.newHttpClient(); HttpRequest request = HttpRequest.newBuilder() .uri(URI.create("https://api.orange.com/{api}/vM/{resource}")) .GET() .setHeader("Authorization", "Bearer YOUR_ACCESS_TOKEN") .build(); HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
Example HttpURLConnection
import java.io.IOException; import java.io.InputStream; import java.net.HttpURLConnection; import java.net.URL; import java.util.Scanner; class Main { public static void main(String[] args) throws IOException { URL url = new URL("https://api.orange.com/{api}/vM/{resource}"); HttpURLConnection httpConn = (HttpURLConnection) url.openConnection(); httpConn.setRequestMethod("GET"); httpConn.setRequestProperty("Authorization", "Bearer YOUR_ACCESS_TOKEN"); InputStream responseStream = httpConn.getResponseCode() / 100 == 2 ? httpConn.getInputStream() : httpConn.getErrorStream(); Scanner s = new Scanner(responseStream).useDelimiter("\\A"); String response = s.hasNext() ? s.next() : ""; System.out.println(response); } }
Example jsoup
import java.io.File; import java.io.FileInputStream; import java.io.IOException; import org.jsoup.Connection; import org.jsoup.Jsoup; class Main { public static void main(String[] args) throws IOException { Connection.Response response = Jsoup.connect("https://api.orange.com/{api}/vM/{resource}") .header("Authorization", "Bearer YOUR_ACCESS_TOKEN") .method(org.jsoup.Connection.Method.GET) .ignoreContentType(true) .execute(); System.out.println(response.parse()); } }
Example okHttp
import java.io.IOException; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response; OkHttpClient client = new OkHttpClient(); Request request = new Request.Builder() .url("https://api.orange.com/{api}/vM/{resource}") .header("Authorization", "Bearer YOUR_ACCESS_TOKEN") .build(); try (Response response = client.newCall(request).execute()) { if (!response.isSuccessful()) throw new IOException("Unexpected code " + response); response.body().string(); }
Example node-fetch
import fetch from 'node-fetch'; fetch('https://api.orange.com/{api}/vM/{resource}', { headers: { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN' } });
Example http
import https from 'https'; const options = { hostname: 'api.orange.com', path: '/{api}/vM/{resource}', headers: { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN' } }; const req = https.get(options, function (res) { const chunks = []; res.on('data', function (chunk) { chunks.push(chunk); }); res.on('end', function () { const body = Buffer.concat(chunks); console.log(body.toString()); }); });
Example requests
import requests headers = { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN', } response = requests.get('https://api.orange.com/{api}/vM/{resource}', headers=headers)
Example http.client
import http.client conn = http.client.HTTPSConnection('api.orange.com') headers = { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN', } conn.request( 'GET', '/{api}/vM/{resource}', headers=headers ) response = conn.getresponse()
Example response:
json { "data": "PROTECTED_RESOURCE_DATA" }
Learn more
provides you with a step-by-step guide with screen shots to help you get started on Orange Developer
provides you with a complete list of possible errors on Orange Developer APIs and how to troubleshoot them
tool that automatically generates code in various languages